Testing is an important but often neglected part of any Django project. In this tutorial we'll review testing best practices and example code that can be applied to any Django app.
Run tests whenever code is PULLed or PUSHed from the repo and in the staging environment before PUSHing to production. When upgrading to a newer version of Django: upgrade locally, run your test suite, fix bugs, PUSH to the repo and staging, and then; test again in staging before shipping the code. Structure your tests to fit your.
- This runner has been added to Django 1.6 as the default test runner. If you use Django 1.6 or above you don't need this app. An alternative Django TESTRUNNER which uses the unittest2 test discovery from a base path specified in the settings, or any other module or package specified to the test management command - including app tests.
- PyCharm Django Test Runner fix for Django 2.0. From tcunittest import TeamcityTestRunner, TeamcityTestResult. From tcmessages import TeamcityServiceMessages. From pycharmrunutils import adjustdjangosyspath. Utils import getrunner.
Broadly speaking there are two types of tests you need to run:
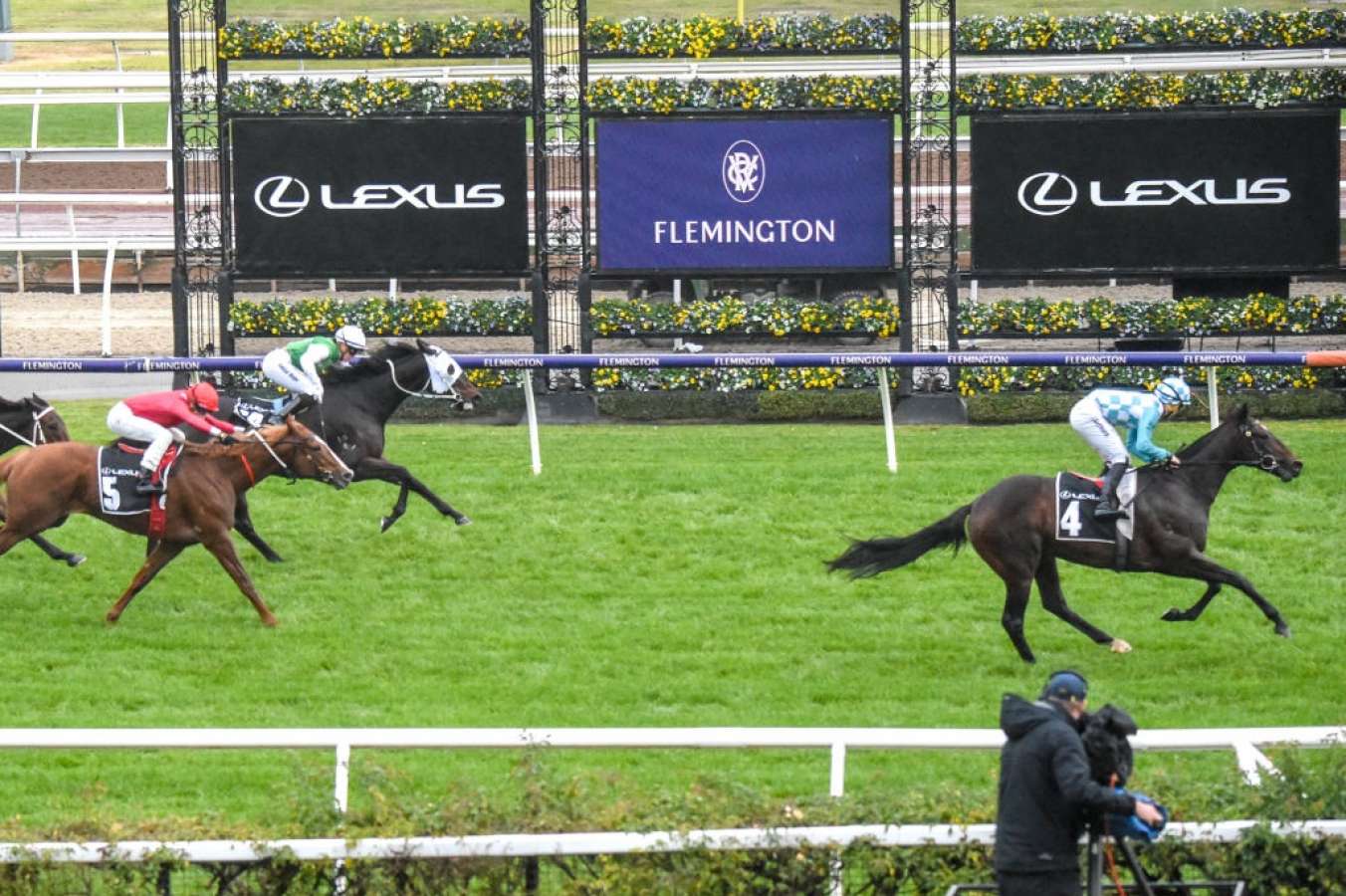
- Unit Tests are small, isolated, and focus on one specific function.
- Integration Tests are aimed at mimicking user behavior and combine multiple pieces of code and functionality.
While we might we use a unit test to confirm that the homepage returns an HTTP status code of 200, an integration test might mimic the entire registration flow of a user.
For all tests the expectation is that the result is either expected, unexpected, or an error. An expected result would be a 200 response on the homepage, but we can--and should--also test that the homepage does not return something unexpected, like a 404 response. Anything else would be an error requiring further debugging.
The main focus of testing should be unit tests. You can't write too many of them. They are far easier to write, read, and debug than integration tests. They are also quite fast to run.
Complete source code is available on Github.
When to run tests
The short answer is all the time! Practically speaking, whenever code is pushed or pulled from a repo to a staging environment is ideal. A continuous integration service can perform this automatically. You should also re-run all tests when upgrading software packages, especially Django itself.
Layout
By default all new apps in a Django project come with a tests.py
file. Any test within this file that starts with test_
will be run by Django's test runner. Make sure all test files start with test_.
As projects grow in complexity, it's recommended to delete this initial tests.py
file and replace it with an app-level tests
folder that contains individual tests files for each area of functionality.
For example:
Sample Project
Let's create a small Django project from scratch and thoroughly test it. It will mimic the message board app
from Chapter 4 of Django for Beginners.
On the command line run the following commands to start our new project. We'll place the code in a folder called testy
on the Desktop, but you can locate the code anywhere you choose.
Now update settings.py
to add our new pages
app and configure Django to look for a project-level templates
folder.
Create our two templates to test for a homepage and about page.
Django Transactiontestcase
Populate the templates with the following simple code.
Update the project-level urls.py
file to point to the pages
app.
Create a urls.py
file within the pages
app.
Then update it as follows:
Django Test Runner Review
And as a final step add our views.
Start up the local Django server.
Then navigate to the homepage at http://127.0.0.1:8000/ and about page at http://127.0.0.1:8000/about to confirm everything is working.
Time for tests.
Django Nose Test Runner
SimpleTestCase
Our Django application only has two static pages at the moment. There's no database involved which means we should use SimpleTestCase.
We can use the existing pages/tests.py
file for our tests for now. Take a look at the code below which adds five tests for our homepage. First we test that it exists and returns a 200
HTTP status code. Then we confirm that it uses the url named home
. We check that the template used is home.html
, the HTML matches what we've typed so far, and even test that it does not contain incorrect HTML. It's always good to test both expected and unexpected behavior.
Now run the tests.
They should all pass.
As an exercise, see if you can add a class for AboutPageTests
in this same file. It should have the same five tests but will need to be updated slightly. Run the test runner once complete. The correct code is below so try not to peak...
Message Board app
Now let's create our message board app so we can try testing out database queries. First create another app called posts
.
Add it to our settings.py
file.
Then run migrate
to create our initial database.
Now add a basic model.
Create a database migration file and activate it.
For simplicity we can just a post via the Django admin. So first create a superuser
account and fill in all prompts.
Update our admin.py
file so the posts
app is active in the Django admin.
Then restart the Django server with python manage.py runserver
and login to the Django admin at http://127.0.0.1:8000/admin/. You should see the admin’s login screen:
Click on the link for + Add
next to Posts
. Enter in the simple text Hello world!
.
On 'save' you'll see the following page.
Now add our views
file.
Create a posts.html
template file.
And add the code below to simply output all posts in the database.
Django Test Suite Runner
Finally, we need to update our urls.py
files. Start with the project-level one located at myproject/urls.py
.
Then create a urls.py
file in the posts
app.
And populate it as follows.
Okay, phew! We're done. Start up the local server python manage.py runserver
and navigate to our new message board page at http://127.0.0.1:8000/posts.
It simply displays our single post entry. Time for tests!
Django Test Runner Pytest
TestCase
TestCase is the most common class for writing tests in Django. It allows us to mock queries to the database.
Let's test out our Post
database model.
With TestCase
the Django test runner will create a sample test database just for our tests. Here we've populated it with the text 'just a test'
.
In the first test we confirm that the test entry has the primary id of 1
and the content matches. Then in the second test on the view we confirm that that it uses the url name posts
, has a 200 HTTP response status code, contains the correct text, and uses the correct template.
Run the new test to confirm everything works.
Next Steps
There is far more testing-wise that can be added to a Django project. A short list includes:
- Continuous Integration: automatically run all tests whenever a new commit is made, which can be done using Github Actions or a service like Travis CI.
- pytest: pytest is the most popular enhancement to Django and Python's built-in testing tools, allowing for more repeatable tests and a heavy use of fixtures.
- coverage: With coverage.py you can have a rough overview of a project's total test coverage.
- Integration tests: useful for testing the flow of a website, such as authentication or perhaps even payments that rely on a 3rd party.
I'm working on a future course on advanced Django testing so make sure to sign up for the LearnDjango newsletter below to be notified when it's ready.
If you need more testing help now, an excellent course is Test-Driven Development with Django, Django REST Framework, and Docker by my friend Michael Herman.